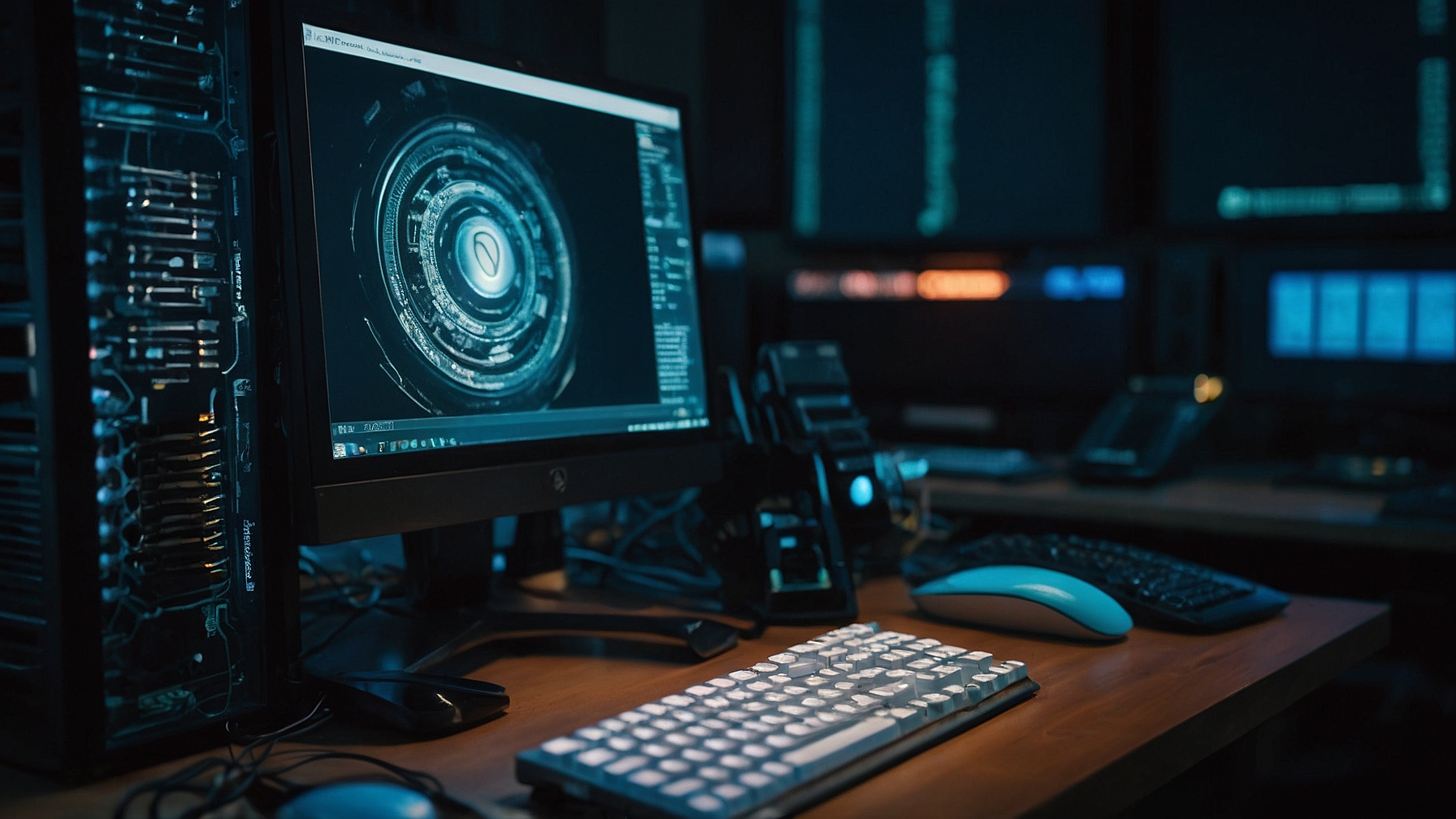
Bash – Read File Line by Line on Linux
Reading a file line by line is a common task in scripting and programming. In Bash, there are several ways to accomplish this. In this guide, we will explore different methods to read a file line by line on Linux.
Method 1: Using the ‘while’ loop
The ‘while’ loop is a powerful construct in Bash that allows us to iterate over each line of a file. Here’s a simple example:
#!/bin/bash
while IFS= read -r line
do
echo "$line"
done < file.txt
In this example, we use the ‘while’ loop to read each line of the ‘file.txt’ file. The ‘read’ command reads a line from the file and assigns it to the ‘line’ variable. We then use ‘echo’ to print the line.
Method 2: Using ‘cat’ and ‘read’ commands
Another way to read a file line by line is by using the ‘cat’ and ‘read’ commands together. Here’s an example:
#!/bin/bash
cat file.txt | while read line
do
echo "$line"
done
In this example, we use the ‘cat’ command to read the contents of the ‘file.txt’ file and pipe it to the ‘read’ command. The ‘read’ command reads each line and assigns it to the ‘line’ variable. We then use ‘echo’ to print the line.
Method 3: Using ‘IFS’ and ‘readarray’ commands
The ‘IFS’ (Internal Field Separator) and ‘readarray’ commands can also be used to read a file line by line. Here’s an example:
#!/bin/bash
IFS=$'\n' readarray -t lines < file.txt
for line in "${lines[@]}"; do
echo "$line"
done
In this example, we set the ‘IFS’ variable to a newline character using the ‘$’\n” syntax. We then use the ‘readarray’ command to read the lines of the ‘file.txt’ file and store them in the ‘lines’ array. Finally, we iterate over each element of the ‘lines’ array using a ‘for’ loop and print each line using ‘echo’.
Similar Commands
Here are some similar commands that can be used to read a file line by line:
sed 's/^.*$/&/' file.txt
: This command uses ‘sed’ to add a line break after each line in the ‘file.txt’ file.awk '{print}' file.txt
: This command uses ‘awk’ to print each line of the ‘file.txt’ file.grep -v '^$' file.txt
: This command uses ‘grep’ to remove empty lines from the ‘file.txt’ file.
Scripts and Ideas
Reading a file line by line can be useful in various scenarios. Here are some script ideas:
- A script that processes a log file and extracts specific information from each line.
- A script that counts the number of lines in a file.
- A script that searches for a specific keyword in a file and prints the matching lines.
- A script that generates a report by processing the contents of a file line by line.
Summary
In this guide, we explored different methods to read a file line by line in Bash on Linux. We learned how to use the ‘while’ loop, ‘cat’ and ‘read’ commands, as well as ‘IFS’ and ‘readarray’ commands. We also discovered some similar commands and discussed script ideas. With these techniques, you can effectively read and process files in your Bash scripts.
Command | Description |
---|---|
while |
A loop construct that allows iterating over each line of a file. |
cat |
A command that reads and concatenates the contents of files. |
read |
A command that reads a line from standard input or a file. |
IFS |
A variable that specifies the field separator used by ‘read’ command. |
readarray |
A command that reads lines from standard input or a file into an array. |
sed |
A command that performs text transformations on input streams. |
awk |
A versatile command-line tool for text processing. |
grep |
A command that searches for patterns in files or input streams. |

This article incorporates information and material from various online sources. We acknowledge and appreciate the work of all original authors, publishers, and websites. While every effort has been made to appropriately credit the source material, any unintentional oversight or omission does not constitute a copyright infringement. All trademarks, logos, and images mentioned are the property of their respective owners. If you believe that any content used in this article infringes upon your copyright, please contact us immediately for review and prompt action.
This article is intended for informational and educational purposes only and does not infringe on the rights of the copyright owners. If any copyrighted material has been used without proper credit or in violation of copyright laws, it is unintentional and we will rectify it promptly upon notification. Please note that the republishing, redistribution, or reproduction of part or all of the contents in any form is prohibited without express written permission from the author and website owner. For permissions or further inquiries, please contact us.