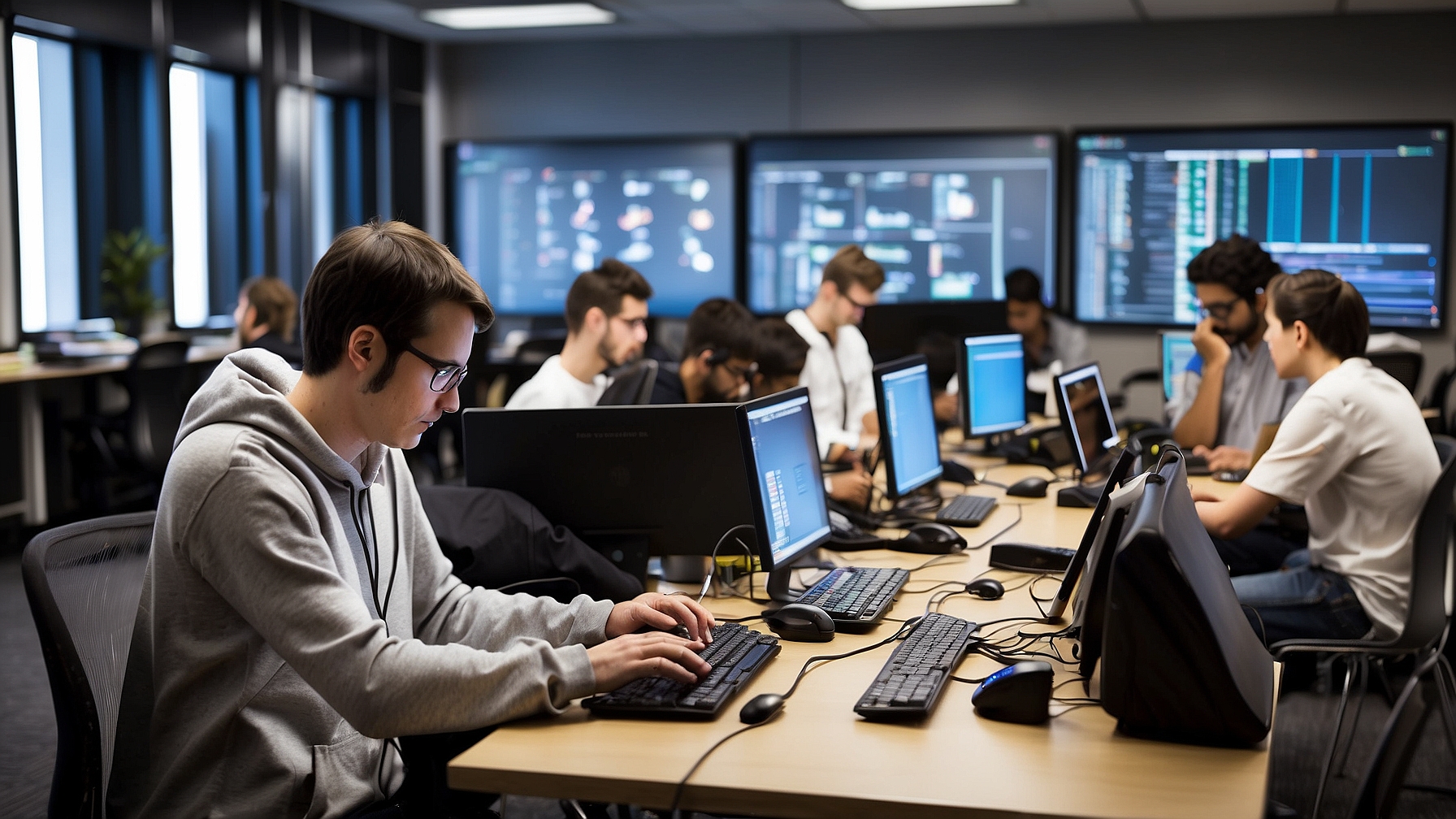
Websocket: Best Practices
Introduction
WebSocket is a communication protocol that provides full-duplex communication channels over a single TCP connection. It enables real-time data transfer between clients and servers, making it ideal for applications that require frequent updates. In this guide, we will discuss some best practices for using WebSockets effectively.
1. Use Secure WebSockets
When implementing WebSockets, it is recommended to use the secure version (wss://) instead of the unsecured version (ws://). Secure WebSockets encrypt the data transmitted between the client and server, ensuring confidentiality and integrity. To establish a secure WebSocket connection, obtain an SSL/TLS certificate for your domain and configure your server accordingly.
2. Implement WebSocket Heartbeats
WebSockets can remain open for extended periods of time, even when there is no data being transmitted. To prevent idle connections from being terminated by firewalls or load balancers, it is advisable to implement WebSocket heartbeats. Heartbeats are small messages sent at regular intervals to keep the connection alive. Both the client and server should have a mechanism to send and respond to heartbeats.
3. Handle WebSocket Errors
WebSocket connections can fail due to various reasons such as network issues, server errors, or timeouts. It is crucial to handle these errors gracefully to ensure a smooth user experience. Implement error handling mechanisms on both the client and server side to handle connection failures, reconnect automatically if possible, and inform the user about any errors that occur.
4. Limit WebSocket Connections
WebSockets can consume server resources, especially when dealing with a large number of concurrent connections. To prevent resource exhaustion, it is recommended to limit the number of WebSocket connections per client or IP address. Implement rate limiting or connection throttling mechanisms to control the number of WebSocket connections and avoid overloading your server.
5. Use Compression
WebSocket messages can be compressed to reduce the amount of data transmitted over the network, resulting in faster and more efficient communication. Enable compression on your WebSocket server and configure the client to support compression as well. Use compression algorithms such as gzip or deflate to compress the payload before sending it over the WebSocket connection.
6. Implement Authentication and Authorization
To secure WebSocket connections, implement authentication and authorization mechanisms. Authenticate clients before establishing a WebSocket connection and authorize them based on their roles and permissions. This ensures that only authorized clients can access the WebSocket server and perform certain actions.
7. Monitor and Log WebSocket Traffic
Monitoring and logging WebSocket traffic can help diagnose issues, identify performance bottlenecks, and track usage patterns. Use logging frameworks or tools to log incoming and outgoing WebSocket messages, including payload size, timestamps, and any relevant metadata. Analyze the logs regularly to gain insights into your WebSocket application’s behavior and performance.
Command Examples
Here are some command examples related to WebSocket implementation:
1. Establishing a WebSocket connection using JavaScript:
const socket = new WebSocket('wss://example.com/socket');
socket.onopen = function() {
console.log('WebSocket connection established.');
};
2. Sending a message over a WebSocket connection:
socket.send('Hello, server!');
3. Handling incoming WebSocket messages:
socket.onmessage = function(event) {
const message = event.data;
console.log('Received message:', message);
};
Similar Commands
Here are some similar commands and concepts related to WebSockets:
Command/Concept | Description |
---|---|
Server-Sent Events (SSE) | A unidirectional communication protocol that allows servers to push data to clients over HTTP or HTTPS. |
Long Polling | A technique where the client sends a request to the server and keeps the connection open until the server has new data to send. |
WebRTC | A real-time communication technology that enables peer-to-peer audio, video, and data sharing in web browsers. |
MQTT | A lightweight messaging protocol designed for resource-constrained devices and unreliable networks. |
Use Cases
WebSockets can be used in a variety of applications, including:
- Real-time chat applications
- Collaborative document editing tools
- Live sports score updates
- Stock market tickers
- Real-time multiplayer games
Ideas for Automation
Here are some ideas for automating WebSocket-related tasks:
- Automatically reconnect WebSocket connections when they fail
- Monitor WebSocket connections and alert administrators if they become unresponsive
- Automatically compress WebSocket messages exceeding a certain size threshold
- Implement automated load testing for WebSocket servers
Scripts for Automation
Here are some example scripts for automating WebSocket tasks:
1. WebSocket reconnection script:
function connectWebSocket() {
const socket = new WebSocket('wss://example.com/socket');
socket.onopen = function() {
console.log('WebSocket connection established.');
};
socket.onclose = function() {
console.log('WebSocket connection closed. Reconnecting...');
setTimeout(connectWebSocket, 5000);
};
}
connectWebSocket();
2. WebSocket monitoring script:
function monitorWebSocket() {
const socket = new WebSocket('wss://example.com/socket');
socket.onopen = function() {
console.log('WebSocket connection established.');
};
socket.onclose = function() {
console.log('WebSocket connection closed. Alerting administrators...');
// Implement alerting mechanism here
};
}
monitorWebSocket();
3. WebSocket message compression script:
function compressMessage(message) {
if (message.length > 1000) {
const compressedMessage = gzip(message);
return compressedMessage;
}
return message;
}
4. WebSocket load testing script:
function simulateWebSocketConnections(numConnections) {
for (let i = 0; i < numConnections; i++) {
const socket = new WebSocket('wss://example.com/socket');
// Perform actions on the WebSocket connection
}
}
simulateWebSocketConnections(100);
Conclusion
Implementing WebSockets requires careful consideration of best practices to ensure secure and efficient real-time communication. By following the recommendations in this guide, you can optimize your WebSocket implementation and provide a seamless user experience. Remember to continuously monitor and improve your WebSocket application to meet the evolving needs of your users.

This article incorporates information and material from various online sources. We acknowledge and appreciate the work of all original authors, publishers, and websites. While every effort has been made to appropriately credit the source material, any unintentional oversight or omission does not constitute a copyright infringement. All trademarks, logos, and images mentioned are the property of their respective owners. If you believe that any content used in this article infringes upon your copyright, please contact us immediately for review and prompt action.
This article is intended for informational and educational purposes only and does not infringe on the rights of the copyright owners. If any copyrighted material has been used without proper credit or in violation of copyright laws, it is unintentional and we will rectify it promptly upon notification. Please note that the republishing, redistribution, or reproduction of part or all of the contents in any form is prohibited without express written permission from the author and website owner. For permissions or further inquiries, please contact us.